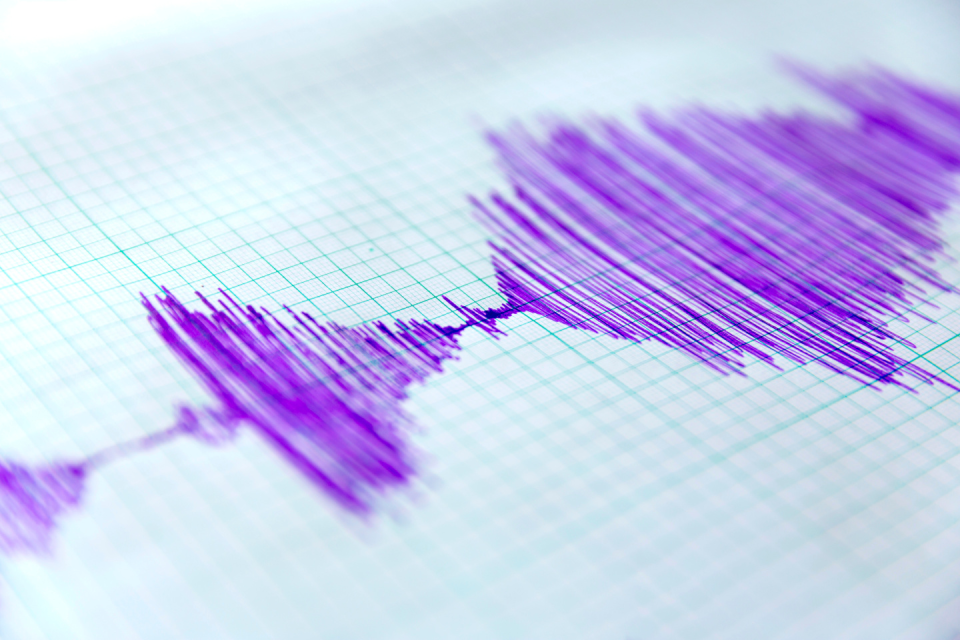
The three pillars of observability are logging, metrics, and tracing:
Logging is having your service emit messages (logs) at specific points in time.
Metrics is making and recording certain measurements of your service at specific points in time.
Tracing is chaining together the calls your service makes, both calls between functions within your service and calls made between your service and others.
These three different ways of observing your service allow you to gain a better perspective of how your service is running and where possible bottlenecks could be. Good observability is also an extremely useful support for data quality.
Today I’m going to talk about the first of the three pillars of observability, logging, at a high level. My goal with this tutorial is to give you a decent starting point for figuring out what’s happening in your application or service. As part of that, I’ll go over a small Python service and a typical route for setting up the logging form of observability.
When writing logs they can end up in different places, such as stdout being piped to your terminal or in a log file that you specify. Since logging to either the terminal or log files can be short-lived, logs can generally be aggregated by a service for longer-term storage.
These log aggregators, paired with parsers and querying services, allow you to better search logs based on the fields they contain.
To create our log, let's start off with a basic Flask app.
Set up a virtual environment to isolate this tutorial if you don't already have one, and then run the following:
1pip install flask
Create a file called app.py with the following content:
1# app.py2
3from flask import Flask4
5
6app = Flask(__name__)7
8
9@app.route("/observability")10def observability():11 return "Hello, Observability!"
Set the environment variable export FLASK_APP=app and run flask run to start the app.
If you now go to localhost:5000/observability in your browser, you should see Hello, Observability!.
Now that we have some scaffolding to work with, let's start logging.
Send it to stdout
For your first logging, you might be tempted to write logs to standard out with print statements, since this is one of the first things that we learn to do in any programming language. That would look something like:
1from flask import Flask2
3app = Flask(__name__)4
5
6@app.route("/observability")7def observability():8